Building Your First AI Agent: A Beginner's Guide
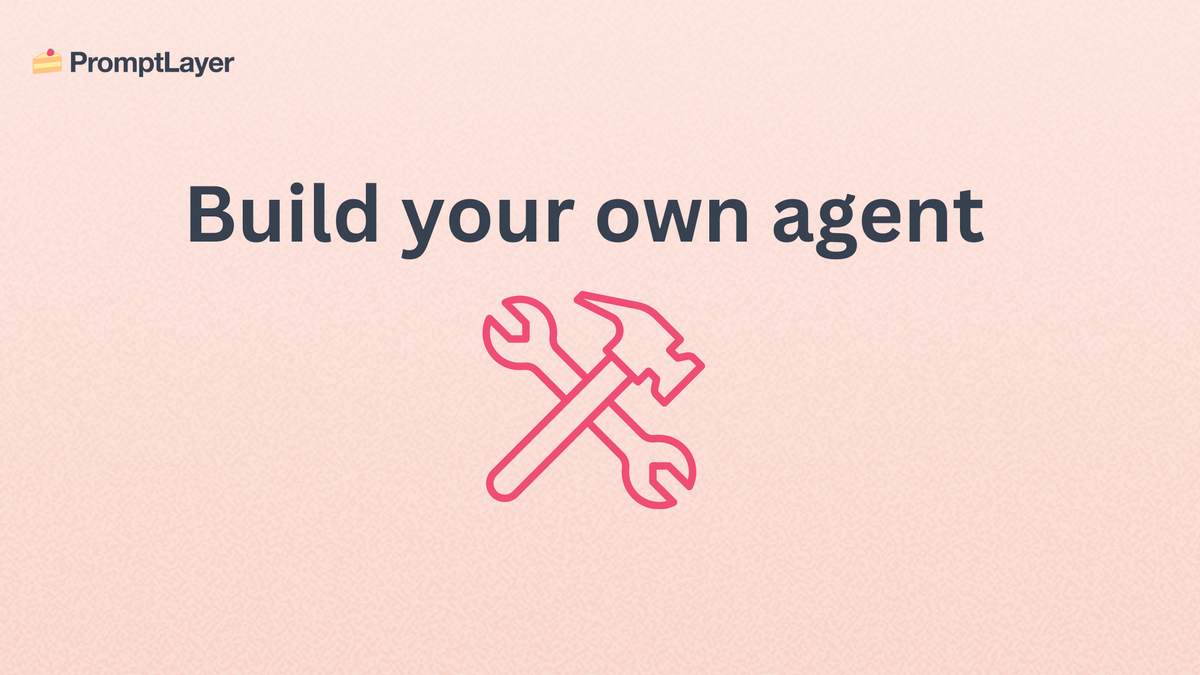
AI agents are programs that can perform tasks, make decisions, and even learn, all with a degree of autonomy. This guide provides a beginner-friendly introduction to the world of AI agents, explaining core concepts and walking you through building your first agent using Python.
Table of Contents
- Introduction
- Basic AI Principles
- Python for AI: Why Python?
- Step-by-Step Guide: Creating an AI Agent
- Practical Project Examples
- Tools and Frameworks to Streamline Development
- Key Takeaways
- Final thoughts
Introduction
An AI agent is a software program that can perceive its environment and act to achieve specific goals autonomously. These agents leverage artificial intelligence techniques to analyze data, make decisions, and automate tasks that would normally require human intelligence. From virtual assistants and game-playing bots to recommendation systems, AI agents are increasingly common. In this guide, we’ll introduce fundamental AI concepts and walk through the process of creating simple AI agents in Python, a beginner-friendly programming language for AI development.
Basic AI Principles
Before diving into coding, it’s important to grasp some basic AI concepts that power intelligent agents:
- Machine Learning (ML): Machine learning is a branch of AI that enables computers to learn from data and improve their performance without explicit programming. Instead of following hard-coded rules, ML algorithms find patterns in example data (training data) and use those patterns to make predictions or decisions. For instance, an ML model can learn to classify emails as “spam” or “not spam” after being trained on many labeled examples.
- Neural Networks & Deep Learning: Neural networks are a set of algorithms modeled loosely after the human brain, designed to recognize patterns in data. A neural network consists of layers of interconnected neurons (nodes) that progressively extract higher-level features from raw input (e.g. detecting edges in an image in early layers, and faces in later layers). Deep learning refers to neural networks with multiple hidden layers (hence “deep”), which can learn complex representations of data. These networks have driven breakthroughs in image recognition, language translation, and more by automatically learning features from large datasets.
- Reinforcement Learning (RL): Reinforcement learning focuses on training an agent that learns by interacting with an environment. The agent takes an action in a given state, and the environment provides feedback in the form of a reward or penalty. Over time, through trial and error, the agent learns a policy (strategy) to maximize its cumulative reward. For example, a game-playing agent might receive +1 reward for winning a game or -1 for losing, and it will gradually learn which actions lead to wins. RL is ideal for problems like game AI, robotics, or any scenario with sequential decisions and a clear goal.
Build agents visually, no coding headaches. Start building today!
Python for AI: Why Python?
Python has become the de facto language for AI development, especially for beginners, due to its simplicity and the rich ecosystem of AI libraries. Its clean syntax and readable code make it easier to experiment with complex algorithms without getting bogged down by programming details. Additionally, Python’s vibrant community has produced powerful libraries that handle the heavy lifting of AI, so you can focus on learning concepts and building agents. Key Python libraries for AI include:
- TensorFlow: An open-source library developed by Google for building and training neural networks. TensorFlow supports efficient computation on CPUs/GPUs and is used for large-scale machine learning and deep learning applications. It provides both low-level flexibility and high-level APIs (like Keras) to design complex models with relative ease.
- PyTorch: An open-source deep learning framework originally created by Facebook (Meta). PyTorch provides a way to build neural networks simply and train them efficiently, with dynamic computation graphs that make debugging and experimentation easier. Known for its flexibility and strong GPU acceleration, PyTorch is widely adopted in both research and industry for developing deep learning models.
- Scikit-learn: A popular library offering a wide range of simple, efficient tools for machine learning and data analysis in Python. Scikit-learn is great for beginners because of its easy-to-use API. It includes many algorithms for classification, regression, clustering, and more – allowing you to quickly train models like decision trees, support vector machines, or k-nearest neighbors on your dataset.
- OpenAI Gym: A Python toolkit that provides a collection of environments (simulated tasks and games) for developing and testing reinforcement learning agents. Gym gives you standardized interfaces for environments like classic control problems (CartPole, MountainCar), Atari games, robotics simulations, etc. This allows you to train your RL agent in a controlled setting and benchmark different algorithms. For example, you can use Gym’s “CartPole” environment to train an agent that balances a pole upright by moving a cart – a classic RL exercise.
Step-by-Step Guide: Creating an AI Agent
Building an AI agent from scratch can be broken down into a series of clear steps. Here’s a step-by-step guide you can follow:
- Define the Problem and Goals: Decide what task your AI agent will perform and what success looks like. Clearly outline the agent’s purpose and the environment it will operate in. For example, is the agent a chatbot that answers customer questions, or a game bot that plays Pac-Man? Defining the goal will guide all other decisions. Also consider the environment’s characteristics: is it deterministic or random? Fully observable or partially observable? By understanding the problem setting, you can choose the right approach (e.g. reinforcement learning for a game, or supervised learning for a classification task).
- Select an Approach and Algorithm: Based on the problem type, choose the AI approach and specific algorithm to use. If you have historical data with correct answers (labels), a supervised learning algorithm (like a neural network or decision tree) might be appropriate. If your agent needs to learn by interaction (no direct correct answers), a reinforcement learning algorithm like Q-learning or policy gradients could be suitable. For example, a chatbot might use a natural language processing model, whereas a game-playing agent might use an RL algorithm. At this stage, also pick the tools/library that support your approach (TensorFlow/PyTorch for neural networks, or OpenAI Gym + an RL library for reinforcement learning, etc.).
- Implement the Agent: Start coding the agent’s logic using Python and the chosen libraries. This involves setting up the model or policy that defines the agent’s behavior. For a neural network–based agent, you would define the network architecture (layers and neurons) using a framework like TensorFlow or PyTorch. For an RL agent, you might write a loop where the agent observes the state from the environment (env.reset() and env.step(...) if using OpenAI Gym) and decides on actions. In this step, you also program the learning part: for ML, define the training routine (how the model updates its weights), and for RL, define how the agent updates its strategy based on rewards (for instance, using the Q-learning update rule or a policy gradient optimizer).
- Train the Agent: Now the agent needs to learn from data or experience. If it’s a machine learning agent, feed it training data and let the model adjust its parameters (weights) to minimize error – this is typically done by calling a training loop or using library functions to fit the model to your data. If it’s an RL agent, run it through many episodes in the environment so it can trial-and-error its way to a good policy. Training might involve thousands of iterations/episodes. Monitor the agent’s progress: for supervised models, watch metrics like accuracy or loss over epochs; for RL agents, observe the reward per episode improving over time.
- Evaluate Performance: After training, evaluate how well your agent performs on its intended task. Use a test dataset (for ML models) or a set of evaluation episodes (for RL agents) that the agent hasn’t seen during training to check for generalization. Measure relevant metrics: accuracy, precision, or recall for prediction tasks; win-rate or average reward for game agents; or simply whether the agent meets the defined success criteria (e.g. does the chatbot answer questions correctly?). If the performance isn’t satisfactory, you may need to return to previous steps – adjust the model, gather more data, or tune hyperparameters (like learning rate, network size, etc.) – and train again. This iterative improvement is a normal part of AI development.
- Deploy or Utilize the Agent: (Optional for beginners) If you’re happy with the agent’s performance, you can integrate it into a real application or simulate it in a real-world scenario. For a simple project, deployment might just mean allowing others to interact with your agent (e.g. running the chatbot in a console or hosting a web demo). Keep monitoring the agent’s behavior; in practical applications, AI agents may require updates or retraining as new data comes in or as goals change.
Practical Project Examples
To solidify your understanding, here are a few beginner-friendly AI agent projects you can try. These projects are manageable in Python and will help you apply the steps above:
- Chatbot Assistant: Create a simple chatbot that can carry on a basic conversation or answer questions. You can start with rule-based responses, or use a library like ChatterBot or a pre-trained language model for more advanced behavior. For example, the ChatterBot library allows you to train a self-learning chatbot with just a few lines of Python, learning to respond based on example dialogues. A chatbot project will introduce you to natural language processing and how AI can understand human language.
- Game-Playing Agent: Build an agent to play a simple game. A classic example is training an agent to play the CartPole game using OpenAI Gym. In CartPole, the agent must balance a pole on a moving cart by applying left or right forces. Using a reinforcement learning algorithm (like Q-learning or Deep Q-Networks), a beginner can train an agent that learns to keep the pole balanced for as long as possible. This project teaches you how agents can learn from interaction and is a fun introduction to RL – you literally see the agent get better at the game over time.
- Automation Bot: Develop a small agent to automate a repetitive task on your computer. For instance, you could code a bot that navigates a website and performs actions (like form-filling or data scraping) or an agent that monitors your email and categorizes urgent messages. While such bots might use simpler logic (not necessarily learning algorithms), you can incorporate AI by, say, using a machine learning model to decide which emails are important. This kind of project shows how AI agents can integrate with real-world applications to save time and effort.
Each of these projects can be approached with the step-by-step method: define the goal (e.g. “play CartPole until the pole balances for 500 timesteps”), pick an approach (maybe use an existing RL library like Stable Baselines3 for CartPole), implement and train, then test the agent’s performance. By starting with small projects, you’ll gain practical experience and confidence to tackle more complex AI agents.
Tools and Frameworks to Streamline Development
Developing AI agents often involves many moving parts – designing models, managing experiments, and iterating on ideas. Fortunately, there are tools and frameworks that can simplify this process:
- PromptLayer Workflows: PromptLayer is a platform that provides a visual, intuitive way to build AI agents, especially those involving language models. Its Workflows feature lets you create agent logic by connecting nodes in a flowchart-like interface (for example, one node could generate a prompt, another could call an AI model, and so on). This node-based workflow reduces the need for extensive coding by allowing you to orchestrate complex AI behaviors graphically. PromptLayer Workflows also make it easy to experiment with different configurations and debug your agent’s decision steps. By abstracting a lot of boilerplate code, they help you focus on the logic and strategy of your AI agent. The benefits include faster iteration, since you can tweak the workflow and re-run it quickly, and easier collaboration, as the visual design is accessible to others on your team. For a beginner, using such a tool can accelerate learning – you can prototype an AI-driven process (like a multi-step chatbot conversation flow or a recommendation system pipeline) without writing every line from scratch.
- Other Helpful Frameworks: Depending on the type of agent, you might explore additional frameworks. For example, Stable Baselines3 (a high-level library for RL) can provide ready-to-use implementations of algorithms like DQN or PPO, so you can train game agents with just a few lines of code. LangChain is a framework for building agents that rely on large language models (great for complex chatbots or text-based decision agents). If your agent involves computer vision, libraries like OpenCV can be useful for processing images. While these are more advanced, knowing they exist is useful as you progress. Most importantly, these tools handle a lot of the “plumbing” of AI tasks, letting you focus on the creative part of agent building.
By leveraging such tools and frameworks, you can build sophisticated AI agents more efficiently. As you gain experience, you’ll naturally mix and match coding your own solutions with using frameworks – the key is understanding the core principles (which you’ve learned above) so you know what the tool is doing for you.
Key Takeaways
- AI Agents and Autonomy: An AI agent is a program that perceives its environment and makes decisions to achieve goals, operating with a degree of autonomy. Even simple agents can automate tasks or make intelligent choices without constant human guidance.
- Core Concepts: Machine learning enables agents to learn from data, neural networks (deep learning) allow for recognizing complex patterns, and reinforcement learning lets agents learn optimal behaviors through trial and error in an environment. Understanding these fundamentals helps in choosing the right approach for a given problem.
- Python Ecosystem: Python is popular for AI development due to its simple syntax and powerful libraries. Tools like TensorFlow, PyTorch, Scikit-learn, and OpenAI Gym provide building blocks for creating and training AI agents, so you don’t have to reinvent the wheel when implementing algorithms.
- Structured Development Process: Building an AI agent involves clear steps: define the problem and goals, select a suitable algorithm/approach, implement the agent’s logic, train it on data or through interaction, and evaluate its performance. Breaking the project down into these steps makes the task less daunting and ensures you cover all necessary components (like gathering data for training and setting evaluation criteria).
- Practice and Iteration: Start with small projects like a chatbot or game agent to apply what you’ve learned. Each project will teach you new skills (e.g. handling text input, or integrating an agent with an environment). Be prepared to iterate – if the agent isn’t performing well, analyze why (maybe you need more training data, or a different model architecture) and improve it. This trial-and-error mindset is part of the AI development process, even for experts.
Final thoughts
Creating AI agents is an exciting journey that combines programming with creative problem-solving. Remember, the key is to keep learning and experimenting. Don’t hesitate to explore tutorials, use tools like PromptLayer’s Workflows to simplify development, and most importantly, have fun with the process. With practice, you’ll be able to design and develop increasingly sophisticated AI agents, and who knows – one day your project might be the intelligent system that others read about in guides like this!
About PromptLayer
PromptLayer is a prompt management system that helps you iterate on prompts faster — further speeding up the development cycle! Use their prompt CMS to update a prompt, run evaluations, and deploy it to production in minutes. Check them out here. 🍰